Navigating unstructured data with MongoDB and Cody
If you're a developer, you may have experienced the overwhelming responsibility of managing a large collection of information. For instance, imagine you have a database that holds tens of thousands of movies. Each movie has its own set of attributes, such as title, description, release year, cast, awards, and genre.
Different stakeholders want to use this data in different ways. For example, you may need to display the movie information on your streaming website, or the data team may want to run an analysis to see which actor groupings gross higher at the box office, and everything in between.
How can you efficiently structure such a vast dataset, while ensuring that your queries are performant, accurate, and can scale as your dataset and use cases grow?
MongoDB is a time-tested powerhouse for handling unstructured, semi-structured, and fully structured data. In this post, we'll take a look at the MongoDB document model, how to query it, the challenges and strengths of working with unstructured data, and how an AI coding assistants like Cody can revolutionize your development workflow.
The MongoDB document model
MongoDB's document model represents a substantial departure from traditional relational database systems. Instead of normalizing your data into tables, which can be difficult to scale and complex to query as your data needs change, MongoDB stores data in flexible, JSON-like documents called BSON (Binary JSON). This approach offers several advantages:
- The schema is more flexible: Unlike rigid table structures in relational databases, MongoDB allows each document to have a unique structure. This is particularly useful for datasets where attributes may vary significantly between entries.
- The data structures are nested: Documents can contain complex nested structures, such as arrays and sub-documents. This allows for a more natural representation of hierarchical or complex data.
- It's easier to scale: The document model facilitates horizontal scaling through sharding, allowing MongoDB to handle massive datasets across distributed systems.
Let's look at a document from a sample movie database called mflix:
This single document encapsulates a wealth of information about the movie Inception, demonstrating the power and flexibility of MongoDB's document model. In a traditional RDBMS, this information would very likely be spread across multiple tables, such as movies, awards, cast, and so on. Combining all of these tables to get the above view would require a complex query consisting of multiple JOINs that could get computationally expensive. With MongoDB's query engine, you can access all of this data effortlessly.
The power of MongoDB queries
Working with your MongoDB data is done through the MongoDB query language. The query language's capabilities are immensely powerful while the developer experience is simple and easy to pick up and master. MongoDB offers the base CRUD operators you'd expect but also boasts an array of complex operators to work with queries, geospatial data, text search, and if that's not enough, a powerful aggregation engine to process your MongoDB data in any way you see fit.
Let's look at a simple query that demonstrates some of these capabilities. We'll assume you are using the MongoDB Node.js driver that you have already set up in a function like this:
For our first MongoDB query, let's write a function to retrieve a list of all the movies that contain the word "Cody" in the title:
We used the .find()
method and searched the title key for a regular expression that contained the word "Cody." We got a few results back, such as Agent Cody Banks and Fetching Cody.
Let's do a more complex query. I want to retrieve the list of movies with an IMDb rating greater than 9 that have only won a single award. I'll ask Cody to generate this query for us.
If we run this function, we will only get one movie that matches this criteria: Hollywood, from our mflix dataset. Cody can be an awesome pair programmer when it comes to generating MongoDB queries as the syntax can get complex quickly.
Let's take a look at another example. MongoDB has a powerful aggregation framework that allows you to transform your data through as many stages as you need to get the outcome you want. Writing aggregation queries can quickly get unwieldy. For example, let's take a look at a MongoDB aggregation pipeline query and try to understand what it does.
This aggregation pipeline finds drama/thriller movies from 2000 to 2020. Then, it calculates the number of such movies and the average rating for each actor, sorting the results to find the top 10 actors in the category. The results can be seen here:
Leveraging Cody for MongoDB
As databases grow in complexity and scale, AI-powered coding assistants become more valuable for developers than ever before. Cody can significantly enhance your MongoDB development experience in a number of ways:
With its autocomplete feature, Cody can help you construct syntactically correct queries. This reduces errors and saves you time on consulting documentation. Cody will infer what you are trying to do based on the code around it, but sometimes it can be helpful to also give Cody guidance in the form of comments.
By analyzing your query and gaining a better understanding of your data model, Cody will also suggest ways to better optimize your queries to improve their performance.
Cody not only provides helpful feedback on potential issues with your queries but also offers alternatives to help you improve your query performance and efficiency.
Practical examples with Cody
Let's take a look at some examples of where Cody can assist with common MongoDB tasks:
1. Constructing a complex query
Suppose you want to find all movies directed by Christopher Nolan after 2010 that have won at least one Academy Award. You might tell Cody:
"Cody, help me write a MongoDB query to find movies directed by Christopher Nolan after 2010 that have won at least one award."
Cody might respond with:
2. Optimizing a query
You might then ask Cody to optimize this query:
"Cody, how can I optimize this query for better performance?"
The current query is:
And what it does is return a list of movies in descending order based on the delta between the Rotten Tomatoes viewer and critic score.
Cody could suggest:
Cody would explain that this compound index covers all fields used in the query, potentially improving performance significantly.
3. Deciphering aggregation pipelines
Developers rarely work in silos and you may need to look at another developer's aggregation pipelines. Depending on the use case, you could spend a great deal of time trying to understand what an aggregation pipeline does, or you can ask Cody and get an overview in seconds.
Let's assume you have this aggregation pipeline:
Trying to read through it to figure out what it's doing can be cumbersome. Asking Cody, we get:
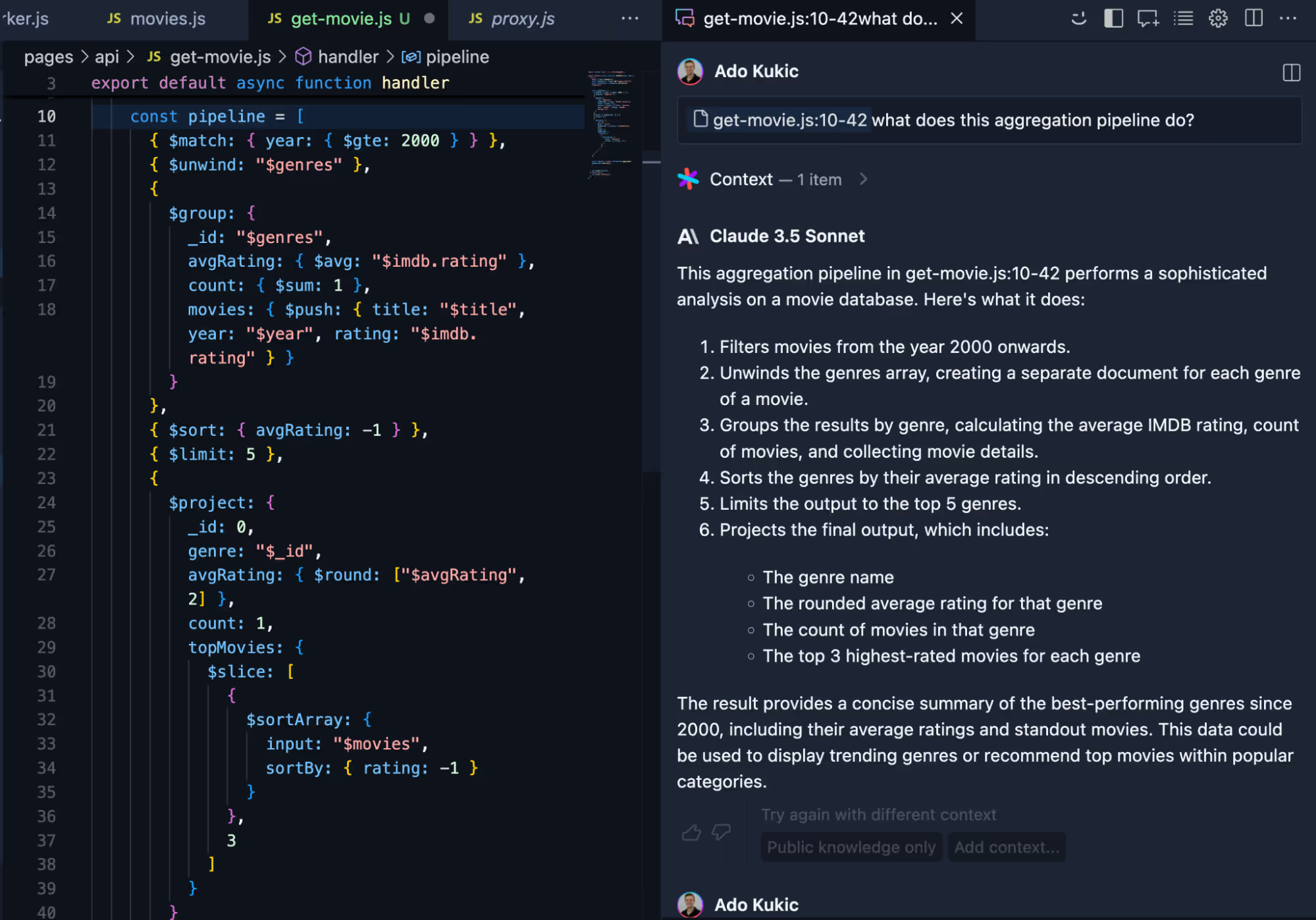
Conclusion
MongoDB's document model offers unparalleled flexibility when it comes to storing and querying unstructured data. While this power does introduce some degree of complexity, Cody is completely changing how developers interact with databases. By leveraging Cody, you can navigate the intricacies of MongoDB queries with greater ease and efficiency, unlocking the full potential of your data.
With practice and Cody by your side, you'll be writing sophisticated, performant queries that extract powerful insights from your data, no matter how unstructured or complex it may be.
Want to learn more from MongoDB? Head to Developer Center to read their latest tutorials, or visit the Developer Community to see what other people are building. Want to chat about how to get the most out of Cody instead? Join our Discord.